Debugging JavaScript applications with console.log
is standard practice, but as our applications grow, the console can quickly become cluttered and hard to read. One way to enhance readability and make specific logs stand out is by using styled console messages.
Why Style Console Logs?
Styled console logs help in:
- Identifying Key Messages Quickly: Highlight critical messages, such as warnings or errors, for quick identification.
- Differentiating Log Types: Use unique styles for different types of messages, like API responses, errors, and debugging checkpoints.
- Making Debugging Collaborative: Shared styling patterns make it easier for team members to locate specific information in the console.
Styling Console Logs with %c
in JavaScript
JavaScript’s console.log
function supports %c
formatting, allowing you to apply CSS styles to log messages. This makes important logs pop out visually.
Here’s an example:
console.log("%c Response Headers:", "background-color:red;color:white;font-size:20px");
Output:
Response Headers:
Creative Uses of Styled Console Logs
Categorizing Logs by Severity
Separate messages by severity, using colors for Success (Green), Warning (Orange), and Error (Red). For example:
console.log("%cSuccess:", "color: green; font-weight: bold;");
console.log("%cWarning:", "color: orange; font-weight: bold;");
console.log("%cError:", "color: red; font-weight: bold;");
Output:
Success:
Warning:
Error:
Tracking Performance with Timestamps
Highlight performance logs to quickly measure and compare execution times:
console.time("fetchData");
// fetch or process data here
console.timeEnd("fetchData");
// Output styled timestamp message
console.log("%cData fetch time:", "color: blue; font-size: 16px; font-weight: bold;");
Using Emoji Indicators
Emojis can serve as visual indicators for easier scanning. For example:
console.log("%c✅ Data loaded successfully!", "color: green;");
console.log("%c⚠️ Warning: Check API response format", "color: orange;");
console.log("%c❌ Error: Data fetch failed!", "color: red; font-weight: bold;");
Output:
✅ Data loaded successfully!
⚠️ Warning: Check API response format
❌ Error: Data fetch failed!
Grouping Console Logs
JavaScript’s console.group()
and console.groupCollapsed()
methods organize related logs in collapsible groups. Adding styles to these groups can further enhance readability.
console.group("%cUser Data Fetch", "color: purple; font-size: 16px;");
console.log("User ID:", user.id);
console.log("User Name:", user.name);
console.groupEnd();
Output:
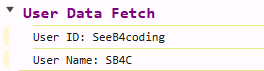
Highlighting Key Steps in Application Flow
For debugging multi-step processes, styled logs can mark checkpoints:
console.log("%cStep 1: Initializing App", "background-color: #008080; color: white; padding: 2px;");
console.log("%cStep 2: Loading Data", "background-color: #0000CD; color: white; padding: 2px;");
console.log("%cStep 3: Rendering UI", "background-color: #008000; color: white; padding: 2px;");
Output:
Step 1: Initializing App
Step 2: Loading Data
Step 3: Rendering UI
Monitoring API Status Codes
Style your logs based on HTTP status codes. For instance:
const status = response.status;
if (status >= 200 && status < 300) {
console.log(`%cSuccess: ${status}`, "color: green; font-weight: bold;");
} else if (status >= 400 && status < 500) {
console.log(`%cClient Error: ${status}`, "color: orange; font-weight: bold;");
} else if (status >= 500) {
console.log(`%cServer Error: ${status}`, "color: red; font-weight: bold;");
}
Emphasizing Important Variables or Objects
For complex data structures, like objects or arrays, you can apply styles to make specific variables stand out.
console.log("%cUser Data:", "font-size: 16px; font-weight: bold; color: darkblue;", userData);
console.log("%cCurrent Settings:", "font-size: 14px; font-style: italic; color: darkgreen;", settings);
Output:
Debugging Network Requests with Headers and Payload
Log request headers, payload, and response in a grouped and styled format for better tracking during API calls:
console.groupCollapsed("%c API Request to /user/profile", "color: blue; font-weight: bold;");
console.log("%cHeaders:", "color: gray;", headers);
console.log("%cPayload:", "color: gray;", payload);
console.groupEnd();
Output:
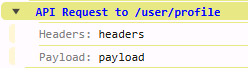
Log-Enhancing with Shadows and Borders
Adding shadows or borders around specific logs can make them visually distinct:
console.log("%cImportant Message", "border: 2px solid red; padding: 4px; box-shadow: 2px 2px 10px rgba(0,0,0,0.5);");
Output:
Highlighting Completion Stages
For async functions or multi-stage processes, use styled logs to mark completed stages:
console.log("%cFetch Step 1 Complete", "background-color: navy; color: white; padding: 2px;");
console.log("%cFetch Step 2 Complete", "background-color: teal; color: white; padding: 2px;");
Output:
Console Style Cheatsheet
Here’s a quick cheatsheet to apply various CSS styles in your console logs:
- Color and Background:
color
,background-color
- Font Size and Weight:
font-size
,font-weight
- Text Decoration:
text-decoration: underline;
- Shadow and Border:
text-shadow
,border
- Spacing:
padding
,margin
Conclusion
With these styled console log techniques, you can make your debugging process not only easier to follow but also more engaging and organized. Styling your logs is an effective way to improve console readability and make crucial information stand out.
Experiment with these techniques to see how they fit into your workflow, and soon, you’ll have a console that’s both visually appealing and highly functional.